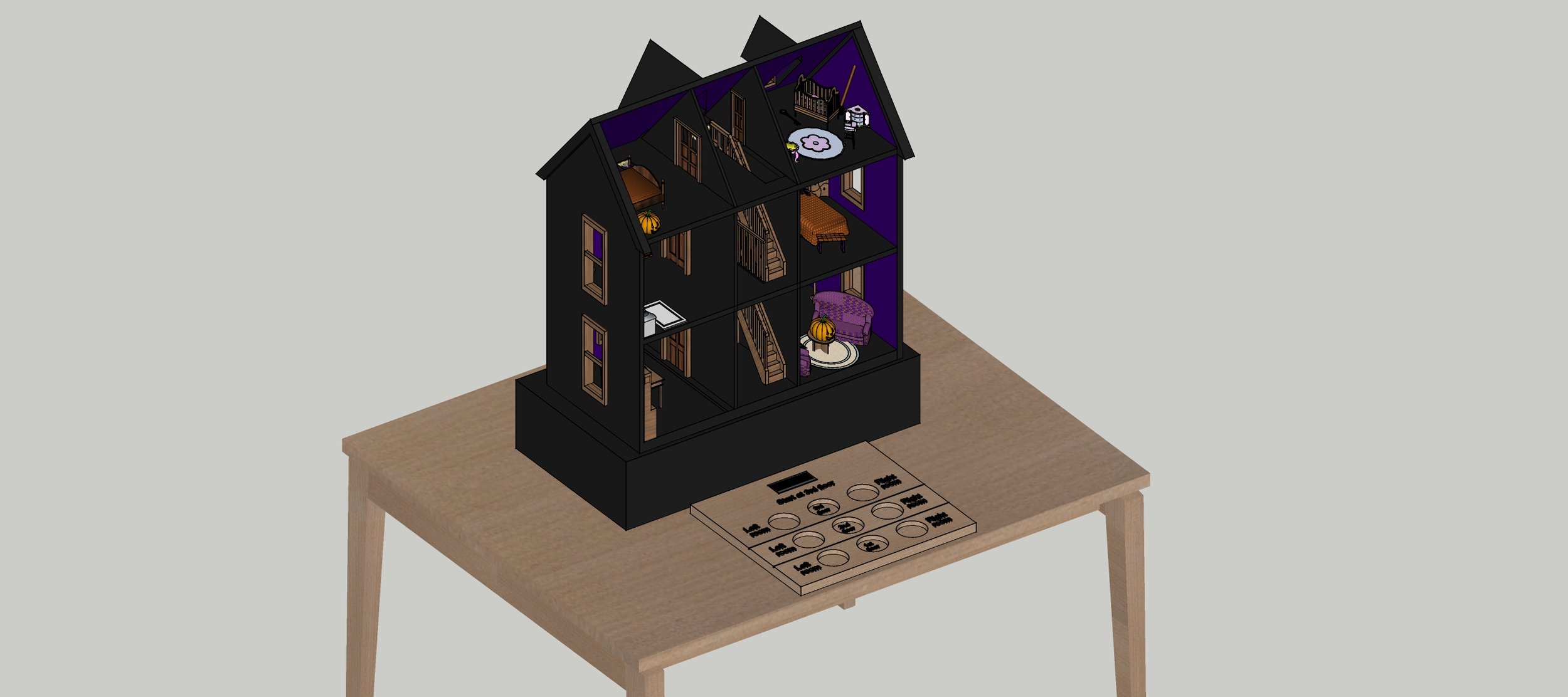
Midterm project
Midterm Project : Design and Prototype
Help Bubble escape a haunted dollhouse
The game involves a 3D figure of Bubble that interacts with the dollhouse using a magnet sensor. The experience includes audio cues, screen prompts, and dynamic lighting effects to create a spooky atmosphere. Note: The game's mechanics rely heavily on user interaction and decision-making, with the goal of finding keys and managing battery life to ultimately determine the character's fate.
This is an interactive game set in a haunted dollhouse featuring characters from the Powerpuff Girls series. The game involves five steps:
Step 1:
Approach the haunted dollhouse.
Activate the journey by placing a bubble figure on a magnet sensor plate.
Encounter various audio, visual, and lighting effects, including scary themes, instructions, and changing lights.
Step 2:
Play a game on the 3rd floor to find a key.
Choose between two rooms with different outcomes: one has a key, the other has a vampire that reduces your battery life.
Interact with Bubble to make progress by placing her on the board.
Step 3:
Move to the 2nd floor with Bubble.
Play a similar game to find a key, again choosing between rooms.
Step 4:
Return to the 1st floor with Bubble and repeat the game to find a key.
Step 5:
Based on the remaining battery life, decide whether Bubble can escape or has to stay in the dollhouse.
Testing Hall effect ( magnet sensor )
Hall Effect ( Magnet sensor)
const int magneticSensorPins[] = {11, 12, 13, 14, 15, 16, 17, 18, 19}; const int ledPins[] = {2, 3, 4, 5, 6, 7, 8, 9, 10}; bool led2On = false; bool led5On = false; bool led8On = false; bool allGroup1On() { return digitalRead(ledPins[0]) && digitalRead(ledPins[1]) && digitalRead(ledPins[2]); } bool allGroup2On() { return digitalRead(ledPins[3]) && digitalRead(ledPins[4]) && digitalRead(ledPins[5]); } void setup() { for (int i = 0; i < 9; i++) { pinMode(magneticSensorPins[i], INPUT_PULLUP); pinMode(ledPins[i], OUTPUT); digitalWrite(ledPins[i], LOW); } Serial.begin(9600); } void loop() { // Print sensor readings for (int i = 0; i < 9; i++) { Serial.print("Sensor "); Serial.print(i + 1); Serial.print(": "); Serial.print(digitalRead(magneticSensorPins[i]) == LOW ? "Triggered" : "Not triggered"); Serial.print(" | "); } Serial.println(); if (digitalRead(magneticSensorPins[1]) == LOW) { digitalWrite(ledPins[1], HIGH); led2On = true; delay(100); // debounce } if (led2On) { if (digitalRead(magneticSensorPins[0]) == LOW) { digitalWrite(ledPins[0], HIGH); delay(100); } if (digitalRead(magneticSensorPins[2]) == LOW) { digitalWrite(ledPins[2], HIGH); digitalWrite(ledPins[0], HIGH); // Turn on LED 1 when LED 3 is on delay(100); } } if (allGroup1On() && digitalRead(magneticSensorPins[4]) == LOW) { digitalWrite(ledPins[4], HIGH); led5On = true; delay(100); } if (led5On) { if (digitalRead(magneticSensorPins[3]) == LOW) { digitalWrite(ledPins[3], HIGH); digitalWrite(ledPins[5], HIGH); // Turn on LED 6 when LED 4 is on delay(100); } if (digitalRead(magneticSensorPins[5]) == LOW) { digitalWrite(ledPins[5], HIGH); delay(100); } } if (allGroup2On() && digitalRead(magneticSensorPins[7]) == LOW) { digitalWrite(ledPins[7], HIGH); led8On = true; delay(100); } if (led8On) { if (digitalRead(magneticSensorPins[6]) == LOW) { digitalWrite(ledPins[6], HIGH); delay(100); } if (digitalRead(magneticSensorPins[8]) == LOW) { digitalWrite(ledPins[8], HIGH); digitalWrite(ledPins[6], HIGH); // Turn on LED 7 when LED 9 is on delay(100); } } }