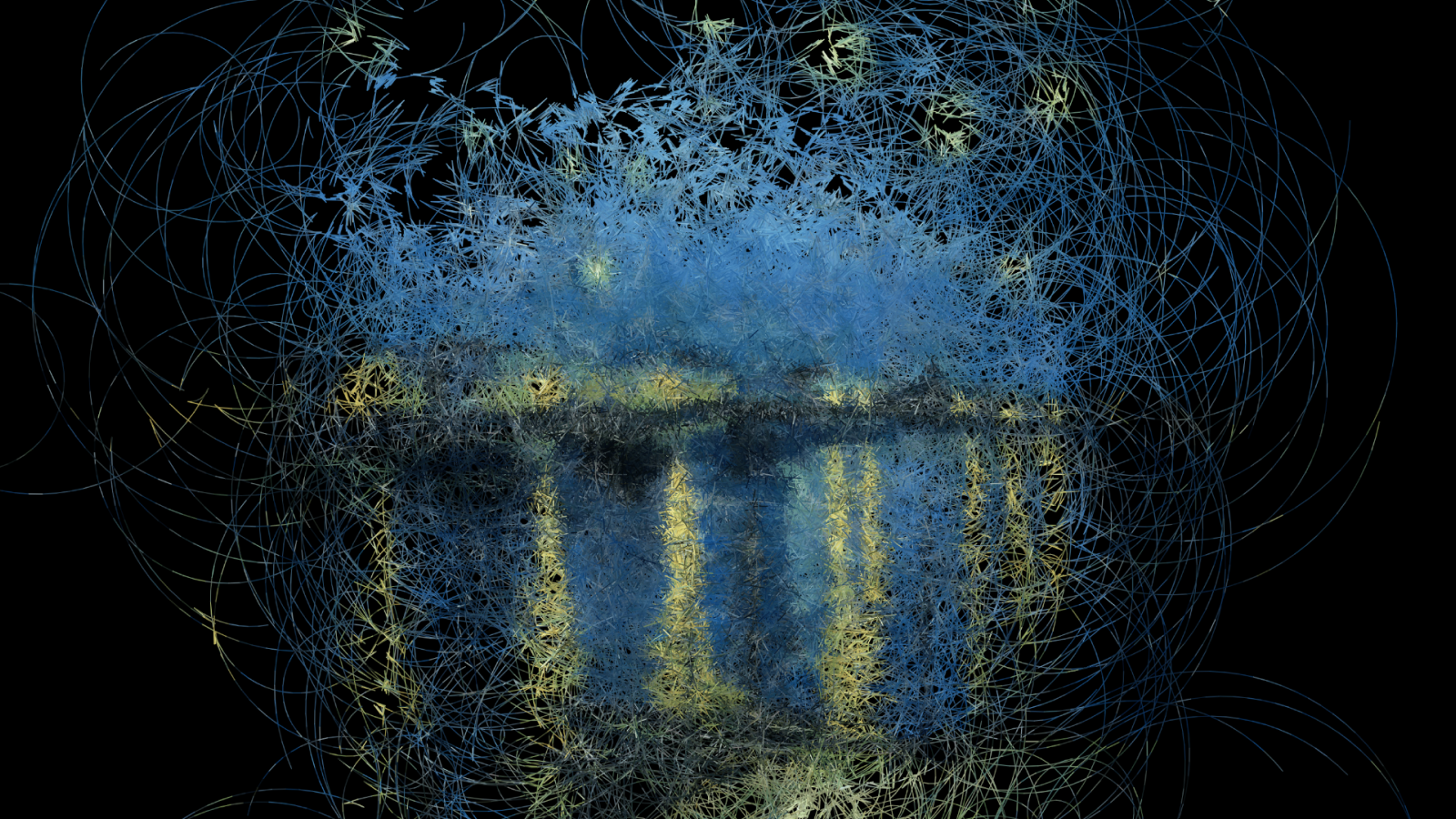
Pixel Painting
Created with Elaine
An interactive sketch where circles leave a trail as they move. The movement appears to be affected by the brightness of background images, and the trails have a color transition effect that's controlled by mouse actions.
Code
function draw() { if (clear) { background(0, 25); // Fading effect by drawing semi-transparent background } for (var i = 0; i < total; i++) { var circle = circles[i]; circle.angle += 1 / circle.radius * circle.dir; circle.pos.x += cos(circle.angle) * circle.radius; circle.pos.y += sin(circle.angle) * circle.radius; if (brightness(img.get(round(circle.pos.x), round(circle.pos.y))) > 70 || circle.pos.x < 0 || circle.pos.x > width || circle.pos.y < 0 || circle.pos.y > height) { circle.dir *= -1; circle.radius = random(3, 15); circle.angle += PI;
function mousePressed() { clear = true; // Start the clearing process transitionProgress = 0; // } function mouseMoved() { if (clear) { clear = false; // Stop clearing the screen when the mouse moves imgIndex = (imgIndex + 1) % imgs.length; // Cycle through images img = imgs[imgIndex]; // Load the next image background(0); // Clear the background fully before drawing the next image }
preload() Function
- loadImage(): This function is called for each of the four star images. They are loaded and stored in the imgs array for later use.
setup() Function
- Sets the current img to the first image in the imgs array.
- createCanvas(): Creates a drawing canvas with the dimensions of the img.
- background(): Sets the initial canvas background to black.
- frameRate(): Sets the number of times the draw() function is called per second.
- Initializes the circles array with 500 objects, each with properties for their previous and current positions, direction, radius, and angle.
draw() Function
- background(): If clear is true, draws a semi-transparent background to create a fading effect.
- Loops over each circle to update its position and draw it
- Updates the circle's angle based on its radius and direction.
- Moves the circle's position in the direction of the angle.
- Checks if the circle is over a bright spot or outside the canvas bounds and inverts its direction if so.
- Grabs the color from the current background image at the circle's position.
- Inverts this color and interpolates between the original and inverted color based on transitionProgress.
- Sets the drawing color to this interpolated color and draws a line from the circle's previous position to its new position.
- Updates the circle's previous position to its current position.
Increments transitionProgress to change the colors over time slowly.
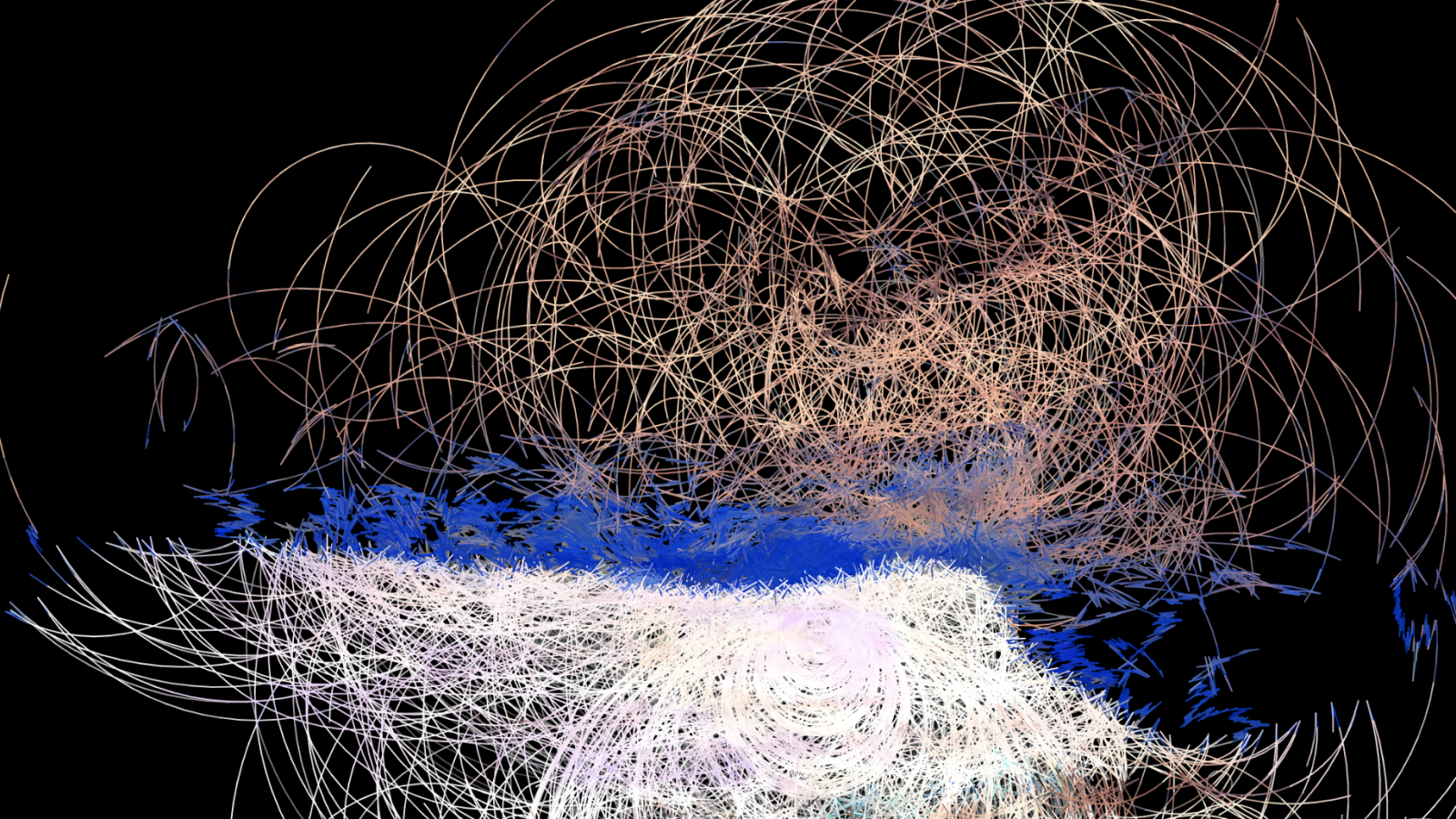
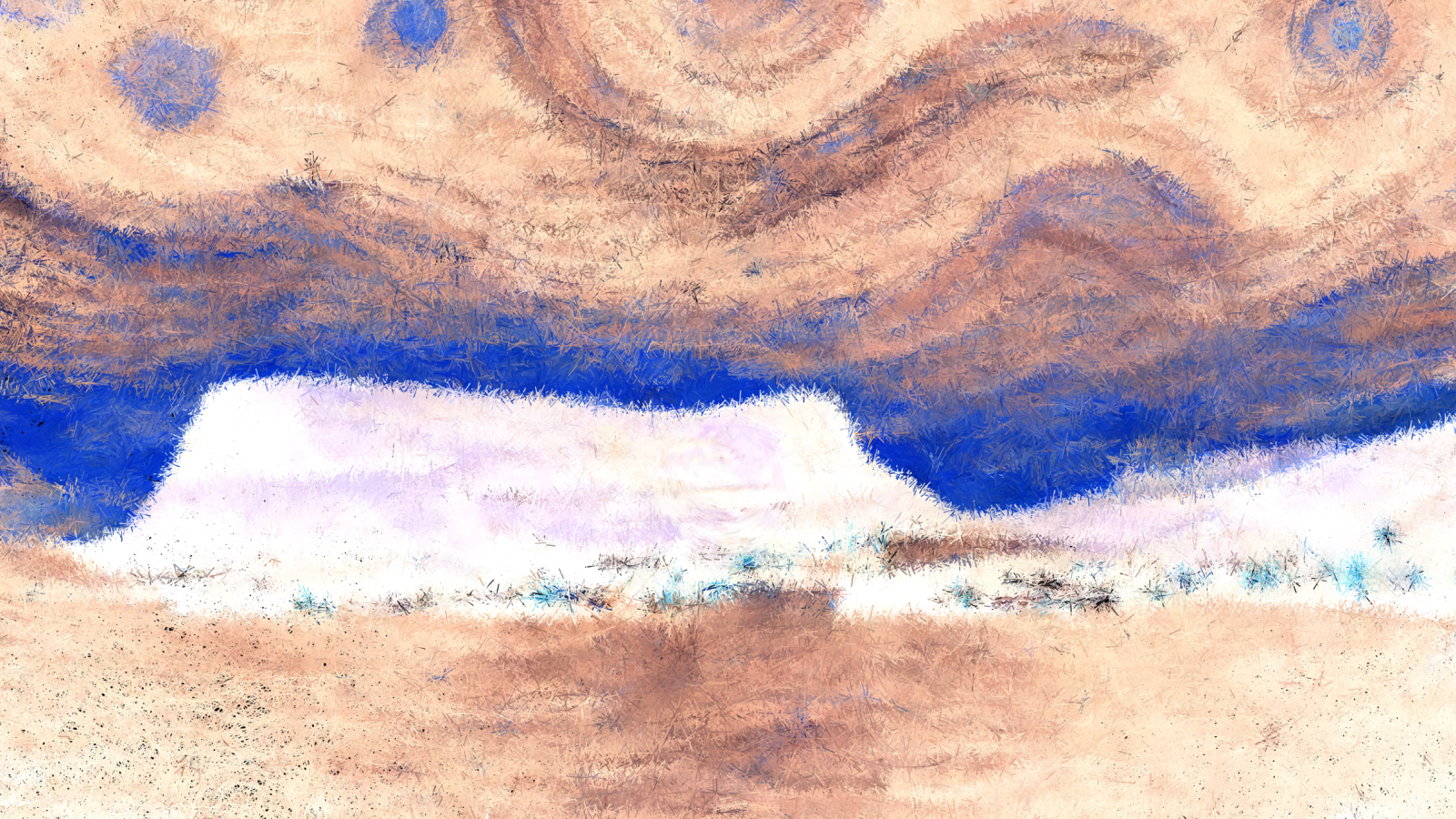
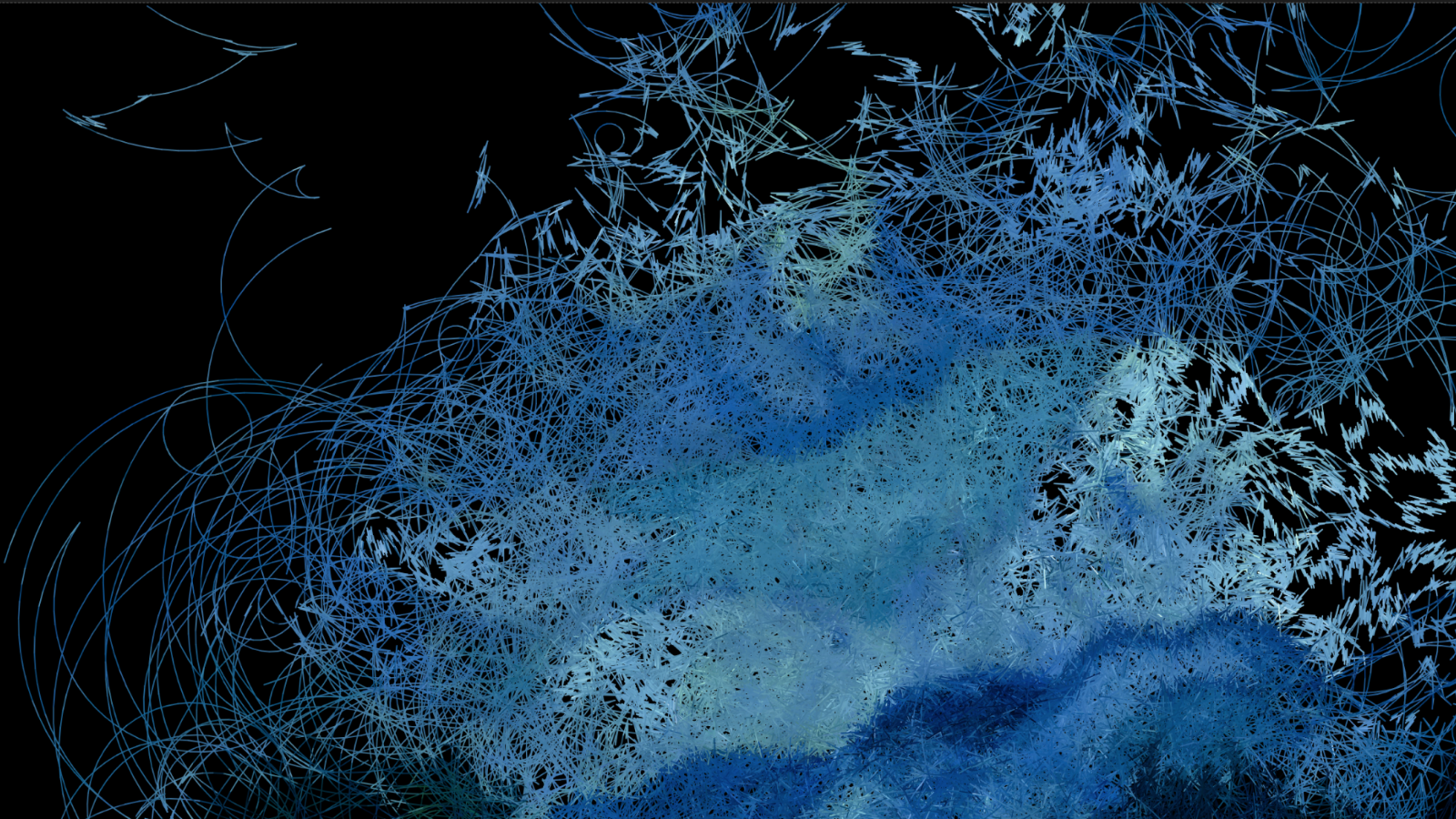
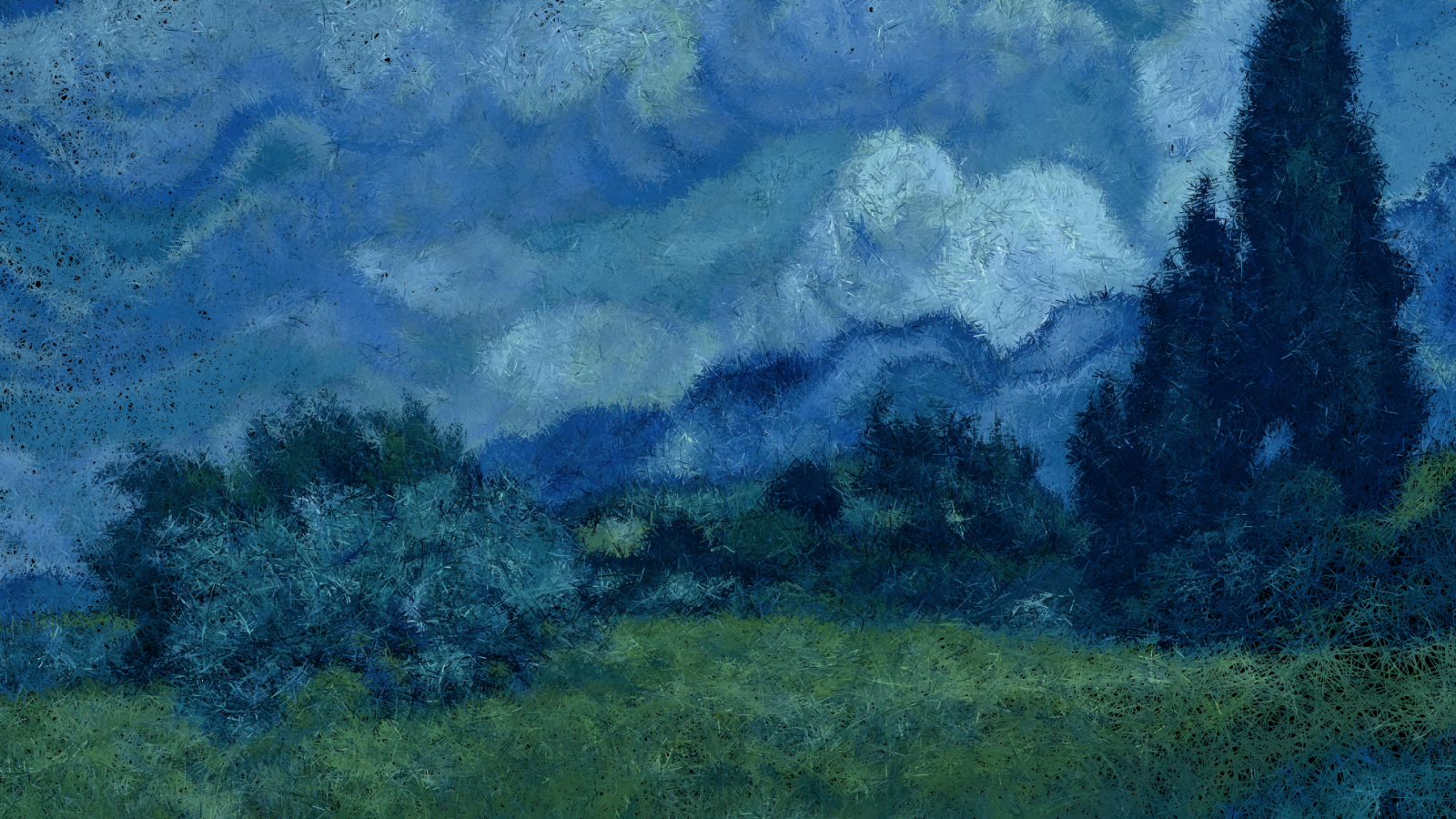
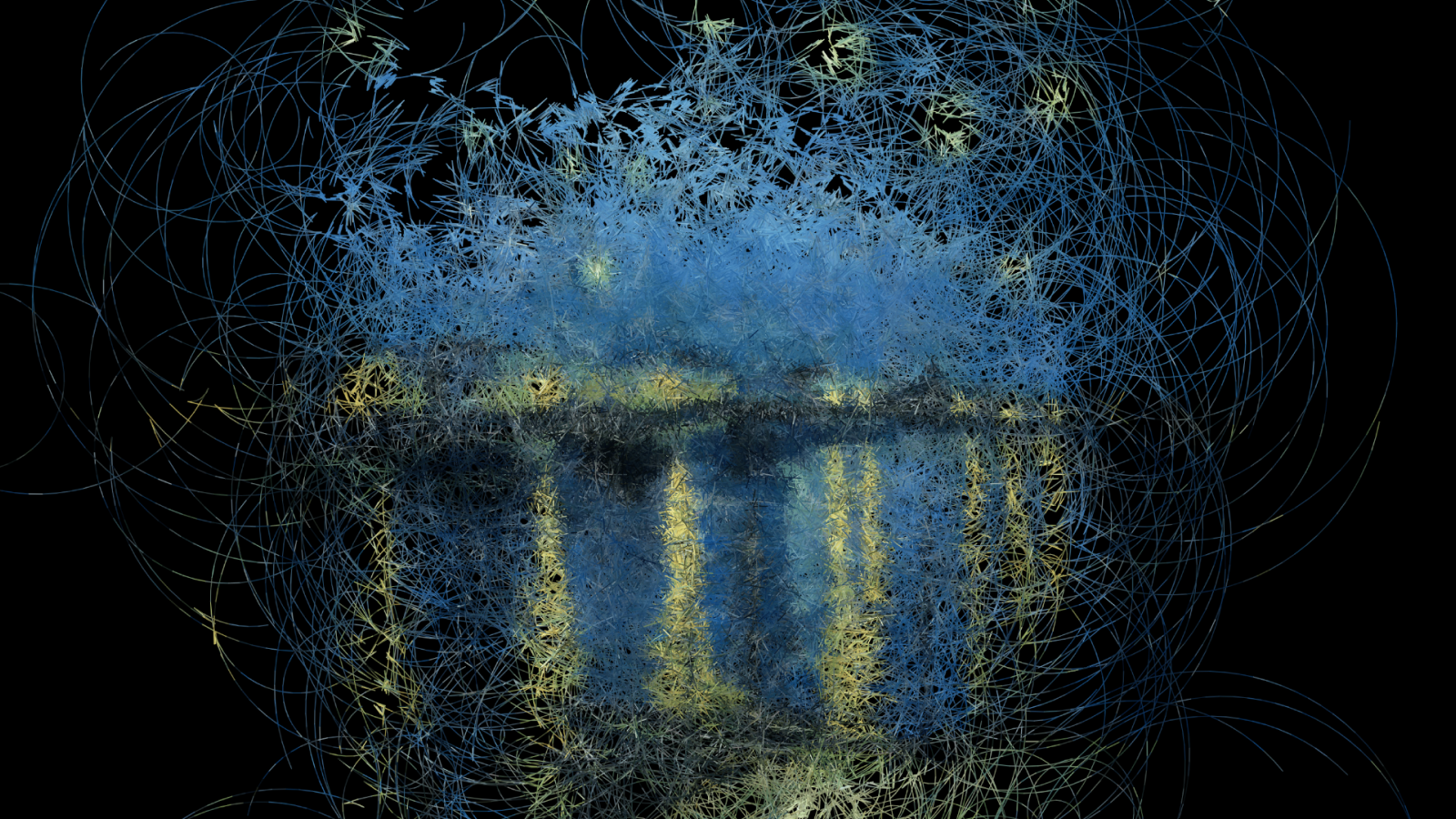
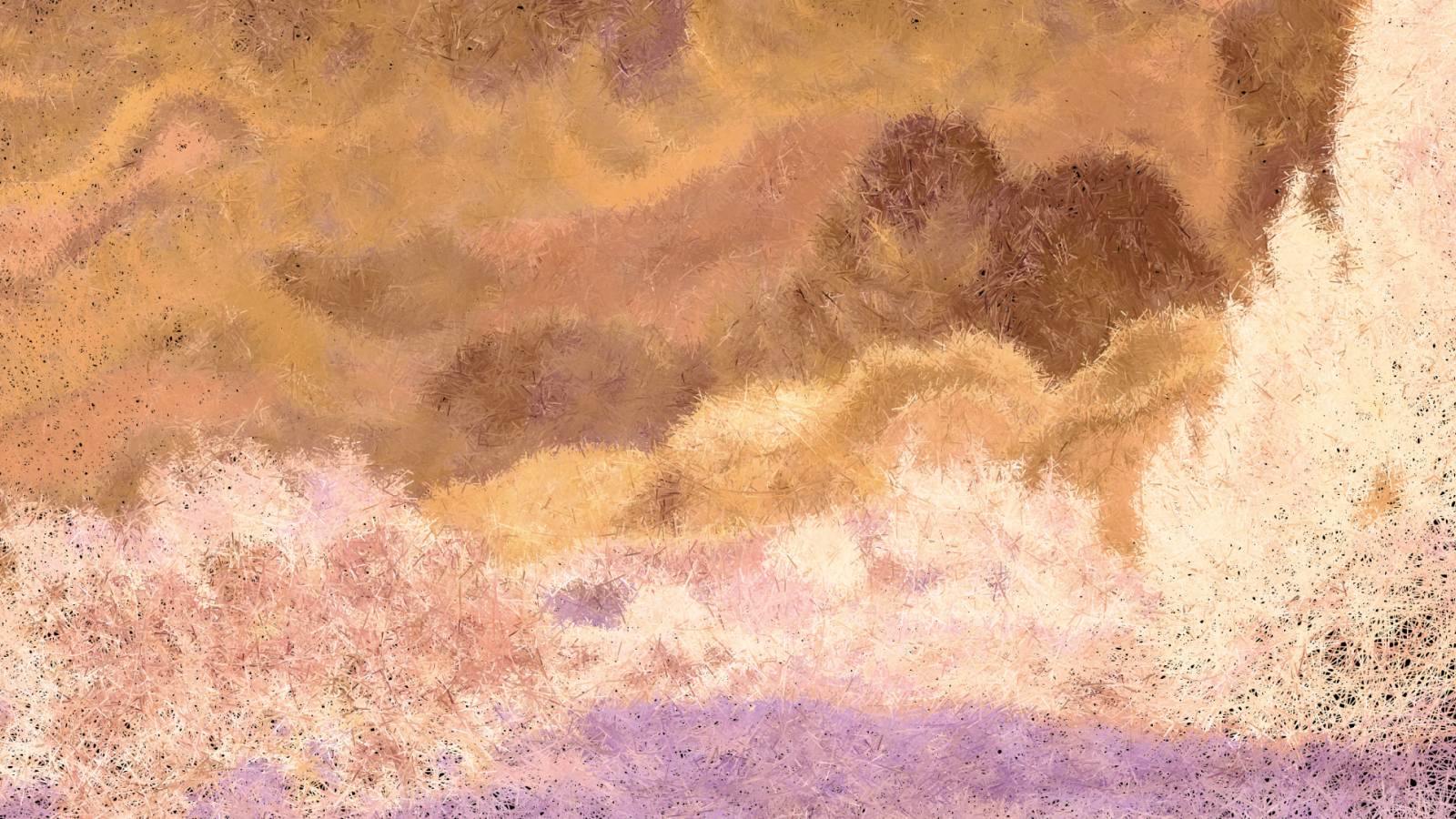